PDF 表格可用于获取调查数据或作为录取表格。鉴于此,本文将教您 如何使用 C++ 创建、填写和编辑可填写的 PDF 表单。
随着计算机和互联网的出现,许多信息都以数字方式捕获。不同的公司想出了解决方案来提高这个过程的效率。一种这样的解决方案是可填写的 PDF 表单。PDF 表单是一种流行的选择,可以轻松地以数字方式捕获信息。PDF 表格可用于获取调查数据或作为录取表格。鉴于此,本文将教您 如何使用 C++ 创建、填写和编辑可填写的 PDF 表单。
- 使用 C++ 创建可填写的 PDF 表单
- 使用 C++ 在 PDF 文件中填写现有表单
- 使用 C++ 修改 PDF 表单中表单域的值
- 使用 C++ 从现有 PDF 表单中删除表单域
使用 C++ 创建可填写的 PDF 表单
在这个例子中,我们将从头开始创建一个带有两个文本框和一个单选按钮的表单。但是,一个文本框是多行的,另一个是单行的。以下是在 PDF 文件中创建表单的步骤。
- 创建Document 类的实例。
- 在文档中添加一个空白页。
- 创建TextBoxField类的实例。
- 设置TextBoxField的属性,如FontSize、Color等。
- 创建第二个TextBoxField的实例并设置其属性。
- 使用Document->get_Form()->Add(System::SharedPtr<Field> field, int32_t pageNumber)方法将两个文本框添加到表单中。
- 创建一个表。
- 创建RadioButtonField类的实例。
- 使用Document->get_Form()->Add(System::SharedPtr<Field> field, int32_t pageNumber)方法将 RadioButton 添加到表单中。
- 创建RadioButtonOptionField类的两个实例来表示单选按钮的选项。
- 设置OptionName、Width和Height并使用RadioButtonField->Add(System::SharedPtr<RadioButtonOptionField> const & newItem)方法将选项添加到单选按钮。
- 使用Cell->get_Paragraphs()->Add(System::SharedPtr<BaseParagraph> 段落)方法将单选按钮的选项添加到表格的单元格中。
- 使用Document->Save(System::String outputFileName)方法保存输出文件。
以下示例代码显示了如何使用 C++ 在 PDF 文件中创建表单。
// Create an instance of the Document classauto pdfDocument = MakeObject();// Add a blank page to the documentSystem::SharedPtrpage = pdfDocument->get_Pages()->Add();System::SharedPtrrectangle1 = MakeObject(275, 740, 440, 770);// Create a TextBoxFieldSystem::SharedPtrnameBox = MakeObject(pdfDocument, rectangle1);nameBox->set_PartialName(u"nameBox1");nameBox->get_DefaultAppearance()->set_FontSize(10);nameBox->set_Multiline(true);System::SharedPtrnameBorder = MakeObject(nameBox);nameBorder->set_Width(1);nameBox->set_Border(nameBorder);nameBox->get_Characteristics()->set_Border(System::Drawing::Color::get_Black());nameBox->set_Color(Aspose::Pdf::Color::FromRgb(System::Drawing::Color::get_Red()));System::SharedPtrrectangle2 = MakeObject(275, 718, 440, 738);// Create a TextBoxFieldSystem::SharedPtrmrnBox = MakeObject(pdfDocument, rectangle2);mrnBox->set_PartialName(u"Box1");mrnBox->get_DefaultAppearance()->set_FontSize(10);System::SharedPtrmrnBorder = MakeObject(mrnBox);mrnBox->set_Width(165);mrnBox->set_Border(mrnBorder);mrnBox->get_Characteristics()->set_Border(System::Drawing::Color::get_Black());mrnBox->set_Color(Aspose::Pdf::Color::FromRgb(System::Drawing::Color::get_Red()));// Add TextBoxField to the formpdfDocument->get_Form()->Add(nameBox, 1);pdfDocument->get_Form()->Add(mrnBox, 1);// Create a tableSystem::SharedPtrtable = MakeObject
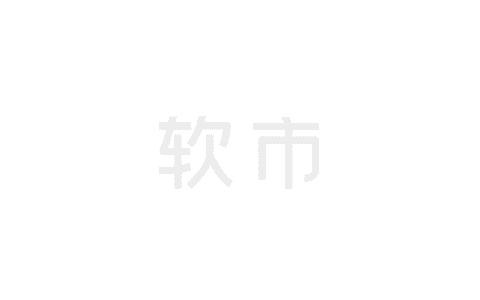
示例代码生成的 PDF 文件的图像
使用 C++ 在 PDF 文件中填写现有表单
在这个例子中,我们将使用上一个例子中生成的文件。我们将使用Document 类加载文件并填充其字段。以下是填写现有 PDF 表单字段的步骤。
- 使用Document 类加载 PDF 文件。
- 使用Document->get_Form()->idx_get(System::String name)方法检索 TextBoxFields 。
- 使用TextBoxField->set_Value(System::String value)方法设置两个 TextBoxField 的值。
- 使用Document->get_Form()->idx_get(System::String name)方法检索RadioButtonField。
- 使用RadioButtonField->set_Selected(int32_t value)方法设置RadioButtonField的值。
- 使用Document->Save(System::String outputFileName)方法保存输出文件。
以下示例代码显示了如何使用 C++ 填充 PDF 文件中的现有表单。
// Load the PDF fileauto pdfDocument = MakeObject<Document>(u"SourceDirectory\Fillable_PDF_Form.pdf");// Retrieve the text box fieldsSystem::SharedPtr<TextBoxField> textBoxField1 = System::DynamicCast<TextBoxField>(pdfDocument->get_Form()->idx_get(u"nameBox1"));System::SharedPtr<TextBoxField> textBoxField2 = System::DynamicCast<TextBoxField>(pdfDocument->get_Form()->idx_get(u"Box1"));// Set the value of the text box fieldstextBoxField1->set_Value(u"A quick brown fox jumped over the lazy dog.");textBoxField2->set_Value(u"A quick brown fox jumped over the lazy dog.");// Retrieve the radio button fieldSystem::SharedPtr<RadioButtonField> radioField = System::DynamicCast<RadioButtonField>(pdfDocument->get_Form()->idx_get(u"radio"));// Set the value of the radio button fieldradioField->set_Selected(1);// Save the output filepdfDocument->Save(u"OutputDirectory\Fill_PDF_Form_Field_Out.pdf");
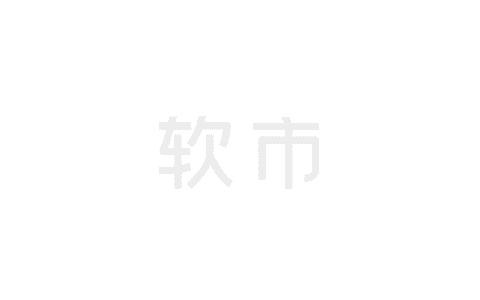
示例代码生成的 PDF 文件的图像
使用 C++ 修改 PDF 表单中表单域的值
使用 Aspose.PDF for C++,我们还可以修改之前填写的字段的值。在这个例子中,我们将使用上一个例子中生成的文件并修改第一个TextBoxField的值。为此,请按照以下步骤操作。
- 使用Document 类加载 PDF 文件。
- 使用Document->get_Form()->idx_get(System::String name)方法检索TextBoxField。
- 使用TextBoxField->set_Value(System::String value)方法更新TextBoxField的值。
- 使用Document->Save(System::String outputFileName)方法保存输出文件。
以下示例代码显示了如何使用 C++ 修改 PDF 表单中字段的值。
// Load the PDF fileauto pdfDocument = MakeObject(u"SourceDirectory\Fill_PDF_Form_Field.pdf");// Retrieve the TextBoxFieldSystem::SharedPtrtextBoxField = System::DynamicCast(pdfDocument->get_Form()->idx_get(u"nameBox1"));// Update the value of the TextBoxFieldtextBoxField->set_Value(u"Changed Value");// Mark the TextBoxField as readonlytextBoxField->set_ReadOnly(true);// Save the output filepdfDocument->Save(u"OutputDirectory\Modify_Form_Field_out.pdf");
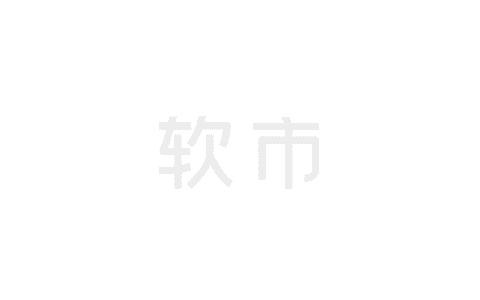
示例代码生成的 PDF 文件的图像
使用 C++ 从现有 PDF 表单中删除表单域
API 还允许您从现有 PDF 表单中删除表单域。以下是从 PDF 表单中删除表单域的步骤。
- 使用Document 类加载 PDF 文件。
- 使用Document->get_Form()->Delete(System::String fieldName)方法删除字段。
- 使用Document->Save(System::String outputFileName)方法保存输出文件。
以下示例代码显示了如何使用 C++ 从现有 PDF 表单中删除表单域。
// Load the PDF fileauto pdfDocument = MakeObject(u"SourceDirectory\Fill_PDF_Form_Field.pdf");// Delete the fieldpdfDocument->get_Form()->Delete(u"nameBox1");// Save the output filepdfDocument->Save(u"OutputDirectory\Delete_Form_Field_out.pdf");
如果你想试用Aspose的全部完整功能,可联系在线客服获取30天临时授权体验。
还想要更多吗可以点击阅读【Aspose最新资源在线文库】,查找需要的教程资源。如果您有任何疑问或需求,请随时加入Aspose技术交流群(),我们很高兴为您提供查询和咨询。
标签:
来源:慧都
声明:本站部分文章及图片转载于互联网,内容版权归原作者所有,如本站任何资料有侵权请您尽早请联系jinwei@zod.com.cn进行处理,非常感谢!