本文展示了如何将Java条码SDK(ZXing和Dynamsoft Barcode Reader)集成到不同的Java应用程序。
Dynamsoft Barcode Reader SDK一款多功能的条码读取控件,只需要几行代码就可以将条码读取功能嵌入到Web或桌面应用程序。这可以节省数月的开发时间和成本。能支持多种图像文件格式以及从摄像机或扫描仪获取的DIB格式。使用Dynamsoft Barcode Reader SDK,你可以创建强大且实用的条形码扫描仪软件,以满足你的业务需求。
Dynamsoft Barcode Reader正式版
本文展示了如何将Java条码SDK(ZXing和Dynamsoft Barcode Reader)集成到不同的Java应用程序。
Java条码应用
在这里,有一个包含多个条形码的图像。
为ZXing和Dynamsoft Barcode Reader配置Maven依赖项:
<repositories> <repository> <id>dbr</id> <url>https://download.dynamsoft.com/maven/dbr/jar</url> </repository></repositories><dependencies> <dependency> <groupId>com.dynamsoft</groupId> <artifactId>dbr</artifactId> <version>7.3</version> </dependency> <dependency> <groupId>com.google.zxing</groupId> <artifactId>core</artifactId> <version>3.4.0</version> </dependency></dependencies>
命令行
创建一个新的Maven项目:
mvn archetype:generate -DgroupId=com.java.barcode -DartifactId=app -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
导入ZXing和Dynamsoft Barcode Reader:
import com.dynamsoft.barcode.*;import com.google.zxing.BinaryBitmap;import com.google.zxing.MultiFormatReader;import com.google.zxing.NotFoundException;import com.google.zxing.RGBLuminanceSource;import com.google.zxing.Result;import com.google.zxing.common.HybridBinarizer;import com.google.zxing.multi.*;
用ImageIO和BufferedImage解码图像文件:
import java.awt.image.*;import javax.imageio.ImageIO;BufferedImage image = null;try { image = ImageIO.read(new File(filename));} catch (IOException e) { System.out.println(e); return;}
测试图像包含多个条形码。要读取使用不同条形码符号编码的多个条形码,我们需要使用MultiFormatReader 和GenericMultipleBarcodeReader。不方便的部分是我们必须先将BufferedImage转换为BinaryBitmap:
BinaryBitmap bitmap = null;int[] pixels = image.getRGB(0, 0, image.getWidth(), image.getHeight(), null, 0, image.getWidth());RGBLuminanceSource source = new RGBLuminanceSource(image.getWidth(), image.getHeight(), pixels);bitmap = new BinaryBitmap(new HybridBinarizer(source)); MultiFormatReader reader = new MultiFormatReader(); GenericMultipleBarcodeReader multiReader = new GenericMultipleBarcodeReader(reader);try { Result[] zxingResults = multiReader.decodeMultiple(bitmap);} catch (NotFoundException e) { e.printStackTrace();}pixels = null;bitmap = null;
调用Dynamsoft Barcode Reader方法要简单得多,因为它支持BufferedImage作为输入参数:
BarcodeReader br = null;try { br = new BarcodeReader("LICENSE-KEY");} catch (Exception e) { System.out.println(e); return;}TextResult[] results = null;try { results = br.decodeBufferedImage(image, "");} catch (Exception e) { System.out.println("decode buffered image: " + e);}
为了方便地运行Java程序,我们可以将所有依赖项组合到一个jar文件中。将maven-assembly-plugin添加到pom.xml:
<build> <plugins> <plugin> <artifactId>maven-assembly-plugin</artifactId> <configuration> <descriptorRefs> <descriptorRef>jar-with-dependencies</descriptorRef> </descriptorRefs> </configuration> </plugin> </plugins></build>
生成Maven项目:
mvn clean install assembly:assembly -Dmaven.test.skip=true
运行Java条码程序,如下所示:
java -cp target/command-line-1.0-SNAPSHOT-jar-with-dependencies.jar com.java.barcode.App ..imagesAllSupportedBarcodeTypes.png
检查SDK性能。我们可以看到ZXing仅返回8个结果,而Dynamsoft Barcode Reader返回18个结果。
GUI
基于上面创建的命令行Java条形码程序,我们可以添加Swing类以将命令行应用程序修改为GUI应用程序。
以下是相关的类:
import javax.swing.JPanel;import javax.swing.JScrollPane;import javax.swing.JTextArea;import javax.swing.JButton;import javax.swing.JFileChooser;import javax.swing.JFrame;import javax.swing.JComboBox;import javax.swing.SwingUtilities;import javax.swing.UIManager;import javax.swing.filechooser.FileNameExtensionFilter;import java.awt.BorderLayout;import java.awt.Toolkit;import java.awt.event.ActionEvent;import java.awt.event.ActionListener;
我们需要的小部件包括JTextArea、JButton、JFileChooser和JComboBox。
- JTextArea:显示结果。
- JButton:触发click事件。
- JFileChooser:从磁盘驱动器中选择一个图像文件。
- JComboBox:切换ZXing和Dynamsoft Barcode Reader。
使用所有小部件初始化布局:
public App() { super(new BorderLayout()); mFileChooser = new JFileChooser(); FileNameExtensionFilter filter = new FileNameExtensionFilter( ".png", "png"); mFileChooser.setFileFilter(filter); mLoad = new JButton("Load File"); mLoad.addActionListener(this); mSourceList = new JComboBox(new String[]{"ZXing", "Dynamsoft"}); mSourceList.setSelectedIndex(0); JPanel buttonPanel = new JPanel(); buttonPanel.add(mSourceList); buttonPanel.add(mLoad); add(buttonPanel, BorderLayout.PAGE_START); mTextArea = new JTextArea(); mTextArea.setSize(480, 480); JScrollPane sp = new JScrollPane(mTextArea); add(sp, BorderLayout.CENTER);}
单击按钮选择图像并解码条形码:
public void actionPerformed(ActionEvent e) { int returnVal = mFileChooser.showOpenDialog(App.this); if (returnVal == JFileChooser.APPROVE_OPTION) { File file = mFileChooser.getSelectedFile(); String filename = file.toPath().toString(); if (mSourceList.getSelectedItem().toString().equals("Dynamsoft")) { TextResult[] results = decodefileDynamsoft(filename); } else { Result[] results = decodefileZXing(filename); } }}
生成并运行GUI应用程序:
mvn clean install assembly:assembly -Dmaven.test.skip=truejava -cp target/gui-1.0-SNAPSHOT-jar-with-dependencies.jar com.java.barcode.App
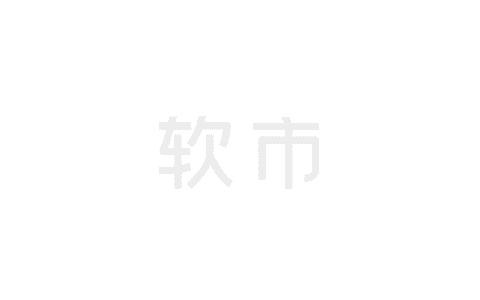
Web
Spring Boot用于创建Web应用程序。我们可以按照官方教程开始使用简单的Web应用程序。
为了快速测试服务器端Java条形码API,我们可以通过添加以下依赖项将swagger-ui集成到Spring Boot应用程序中:
<dependency> <groupId>org.springdoc</groupId> <artifactId>springdoc-openapi-core</artifactId> <version>1.1.45</version> <exclusions> <exclusion> <groupId>io.github.classgraph</groupId> <artifactId>classgraph</artifactId> </exclusion> </exclusions></dependency><dependency> <groupId>org.springdoc</groupId> <artifactId>springdoc-openapi-ui</artifactId> <version>1.1.45</version></dependency>
创建一个包含ZXing和Dynamsoft Barcode Reader的POST映射的控制器:
@RestControllerpublic class BarcodeController { private DynamsoftBarcode mDynamsoftBarcode; private ZXingBarcode mZXingBarcode; @Autowired public BarcodeController(DynamsoftBarcode dynamsoft, ZXingBarcode zxing) { mDynamsoftBarcode = dynamsoft; mZXingBarcode = zxing; } @PostMapping(value = "/api/dynamsoft" , consumes = MediaType.MULTIPART_FORM_DATA_VALUE , produces = MediaType.APPLICATION_JSON_VALUE) public BarcodeResponse getDynamsoft(@RequestPart MultipartFile file) throws Exception { return mDynamsoftBarcode.decode(file.getOriginalFilename(), file.getInputStream()); } @PostMapping(value = "/api/zxing" , consumes = MediaType.MULTIPART_FORM_DATA_VALUE , produces = MediaType.APPLICATION_JSON_VALUE) public BarcodeResponse getZXing(@RequestPart MultipartFile file) throws Exception { return mZXingBarcode.decode(file.getOriginalFilename(), file.getInputStream()); }}
生成并运行Java条码Web应用程序:
mvn clean installjava -jar target/web-1.0-SNAPSHOT.jar
访问http:// localhost:8080 / swagger-ui.html通过POST事件测试ZXing和Dynamsoft条码读取器。
那么Android开发呢/strong>
在使用Java条码SDK开发移动应用程序时,我们使用Gradle而不是Maven添加依赖项。
allprojects { repositories { google() jcenter() maven { url "http://download.dynamsoft.com/maven/dbr/aar" } }}implementation 'com.google.zxing:core:3.4.0'implementation 'com.dynamsoft:dynamsoftbarcodereader:7.3.0'
本文内容到这里就结束了,希望对您有所帮助~您可以下载Dynamsoft Barcode Reader试用版免费评估~
相关内容推荐:
如何在Python中对Dynamsoft Barcode Reader性能进行基准测试
Dynamsoft Barcode Reader教程:如何使用Dynamsoft Java条形码阅读器扫描多个条形码
想要购买该产品正版授权,或了解更多产品信息请点击【咨询在线客服】
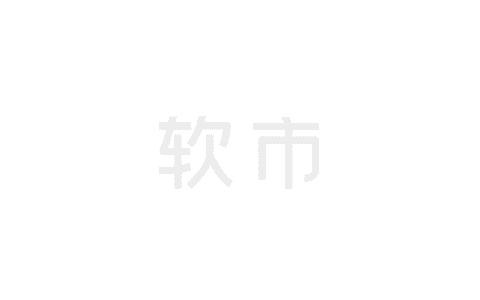
标签:
来源:慧都
声明:本站部分文章及图片转载于互联网,内容版权归原作者所有,如本站任何资料有侵权请您尽早请联系jinwei@zod.com.cn进行处理,非常感谢!