在本文中,您将学习如何使用Spire.Doc for .NET在 C# 和 VB.NET 中合并或拆分 Word 文档中的表格单元格。
合并单元格是指将两个或多个单元格组合成一个较大的单元格,而拆分单元格是指将一个单元格分成两个或多个较小的单元格。在 Microsoft Word 中创建或编辑表格时,您可能经常需要合并或拆分表格单元格以更好地呈现数据。在本文中,您将学习如何使用Spire.Doc for .NET在 C# 和 VB.NET 中合并或拆分 Word 文档中的表格单元格。
一、安装适用于 .NET 的 Spire.Doc
首先,您需要添加包含在 Spire.Doc for.NET 包中的 DLL 文件作为您的 .NET 项目中的引用。DLL 文件可以从此链接下载或通过NuGet安装。
PM> Install-Package Spire.Doc
二、使用 C# 和 VB.NET 在 Word 中合并表格单元格
在 Microsoft Word 中,您可以将两个或多个相邻的单元格水平或垂直合并为一个更大的单元格。在 Spire.Doc 中,您可以使用Table.ApplyHorizontalMerge()或Table.ApplyVerticalMerge()方法实现相同的目的。以下是详细步骤:
- 初始化Document类的一个实例。
- 使用Document.LoadFromFile()方法加载 Word 文档。
- 通过Document.Sections[int]属性通过索引获取文档中的特定部分。
- 使用Section.AddTable()方法将表格添加到该部分。
- 使用Table.ResetCells()方法指定表格的行数和列数。
- 使用Table.ApplyHorizontalMerge()方法水平合并表格中的特定单元格。
- 使用Table.ApplyVerticalMerge()方法垂直合并表格中的特定单元格。
- 向表中添加一些数据。
- 将样式应用于表格。
- 使用Document.SaveToFile()方法保存结果文档。
【C#】
using Spire.Doc;using Spire.Doc.Documents;namespace MergeTableCells{class Program{static void Main(string[] args){//Create a Document instanceDocument document = new Document();//Load a Word documentdocument.LoadFromFile("Input.docx");//Get the first sectionSection section = document.Sections[0];//Add a 4 x 4 table to the sectionTable table = section.AddTable();table.ResetCells(4, 4);//Horizontally merge cells 1, 2, 3, and 4 in the first rowtable.ApplyHorizontalMerge(0, 0, 3);//Vertically merge cells 3 and 4 in the first columntable.ApplyVerticalMerge(0, 2, 3);//Add some data to the tablefor (int row = 0; row < table.Rows.Count; row++){for (int col = 0; col < table.Rows[row].Cells.Count; col++){TableCell cell = table[row, col];cell.CellFormat.VerticalAlignment = VerticalAlignment.Middle;Paragraph paragraph = cell.AddParagraph();paragraph.Format.HorizontalAlignment = HorizontalAlignment.Center;paragraph.Text = "Text";}}//Apply a style to the tabletable.ApplyStyle(DefaultTableStyle.LightGridAccent1);//Save the result documentdocument.SaveToFile("MergeCells.docx", FileFormat.Docx2013);}}}
【VB.NET】
Imports Spire.DocImports Spire.Doc.DocumentsNamespace MergeTableCellsFriend Class ProgramPrivate Shared Sub Main(ByVal args As String())'Create a Document instanceDim document As Document = New Document()'Load a Word documentdocument.LoadFromFile("Input.docx")'Get the first sectionDim section As Section = document.Sections(0)'Add a 4 x 4 table to the sectionDim table As Table = section.AddTable()table.ResetCells(4, 4)'Horizontally merge cells 1, 2, 3, and 4 in the first rowtable.ApplyHorizontalMerge(0, 0, 3)'Vertically merge cells 3 and 4 in the first columntable.ApplyVerticalMerge(0, 2, 3)'Add some data to the tableFor row As Integer = 0 To table.Rows.Count - 1For col As Integer = 0 To table.Rows(row).Cells.Count - 1Dim cell As TableCell = table(row, col)cell.CellFormat.VerticalAlignment = VerticalAlignment.MiddleDim paragraph As Paragraph = cell.AddParagraph()paragraph.Format.HorizontalAlignment = HorizontalAlignment.Centerparagraph.Text = "Text"NextNext'Apply a style to the tabletable.ApplyStyle(DefaultTableStyle.LightGridAccent1)'Save the result documentdocument.SaveToFile("MergeCells.docx", FileFormat.Docx2013)End SubEnd ClassEnd Namespace
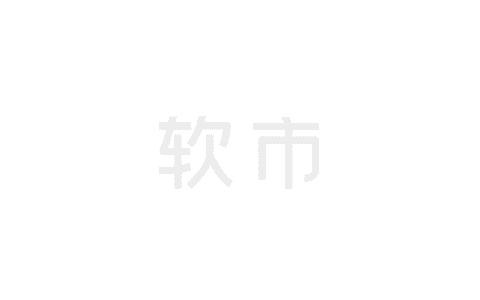
三、使用 C# 和 VB.NET 在 Word 中拆分表格单元格
Spire.Doc for .NET 提供了TableCell.SplitCell()方法,使您能够将 Word 表格中的一个单元格拆分为两个或多个单元格。以下是详细步骤:
- 初始化Document类的一个实例。
- 使用Document.LoadFromFile()方法加载 Word 文档。
- 通过Document.Sections[int]属性通过索引获取文档中的特定部分。
- 通过Section.Tables[int]属性根据其索引获取节中的特定表。
- 通过Table.Rows[int].Cells[int]属性获取要拆分的表格单元格。
- 使用TableCell.SplitCell()方法将单元格拆分为特定数量的列和行。
- 使用Document.SaveToFile()方法保存结果文档。
【C#】
using Spire.Doc;namespace SplitTableCells{class Program{static void Main(string[] args){//Create a Document instanceDocument document = new Document();//Load a Word Documentdocument.LoadFromFile("MergeCells.docx");//Get the first sectionSection section = document.Sections[0];//Get the first table in the sectionTable table = section.Tables[0] as Table;//Get the 4th cell in the 4th rowTableCell cell1 = table.Rows[3].Cells[3];//Split the cell into 2 columns and 2 rowscell1.SplitCell(2, 2);//save the result documentdocument.SaveToFile("SplitCells.docx", FileFormat.Docx2013);}}}
【VB.NET】
Imports Spire.DocNamespace SplitTableCellsFriend Class ProgramPrivate Shared Sub Main(ByVal args As String())'Create a Document instanceDim document As Document = New Document()'Load a Word Documentdocument.LoadFromFile("MergeCells.docx")'Get the first sectionDim section As Section = document.Sections(0)'Get the first table in the sectionDim table As Table = TryCast(section.Tables(0), Table)'Get the 4th cell in the 4th rowDim cell1 As TableCell = table.Rows(3).Cells(3)'Split the cell into 2 columns and 2 rowscell1.SplitCell(2, 2)'save the result documentdocument.SaveToFile("SplitCells.docx", FileFormat.Docx2013)End SubEnd ClassEnd Namespace
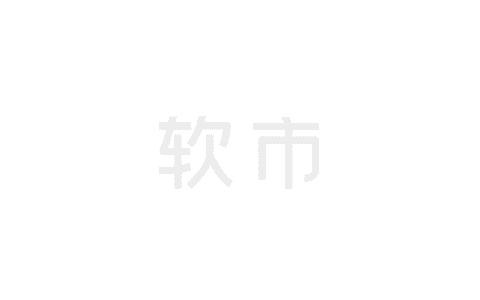
以上便是如何在 Word 中合并或拆分表格单元格,如果您有其他问题也可以继续浏览本系列文章,获取相关教程,你还可以给我留言或者加入我们的官方技术交流群。
欢迎下载|体验更多E-iceblue产品
获取更多信息请咨询在线客服 ;
标签:
来源:慧都
声明:本站部分文章及图片转载于互联网,内容版权归原作者所有,如本站任何资料有侵权请您尽早请联系jinwei@zod.com.cn进行处理,非常感谢!